Hello friends, in this article we have compiled a list of the top 100 core Java interview questions. These questions are designed for both fresher and experienced professionals of software development as well as automation testing profiles. We will start with the questions related to the basics of Java and then move to more complex interview questions.
Ques.1. What is Java?
Ans. Java is an object-oriented programming language, developed in 1995 by Sun Microsystems. It is a computer-based platform and is used to develop applications.
Ques.2. What are some features of Java?
Ans. Some of the major features of Java are-
- It is simple and easy to learn with a simple syntax.
- It is an object-based language. This means it is easier to implement, and can easily be extended.
- The Java platform has null interactions with the OS when a program is run. This makes it very secure as the program is developed without any virus and is tamper-free.
- It is architectural-neutral; this means a compiled code can be run on various processors in the presence of a Java runtime system.
- Programs written in Java can perform various tasks simultaneously. This multi-threaded feature is very helpful in creating interactive applications.
Ques.4. What is JDK?
Ans. JDK means Java Development Kit. This term should not be confused with JRE and JVM. The JDK provides an environment to build, run, and execute programs. All the development tools required to develop a program and the JRE come inside the JDK.
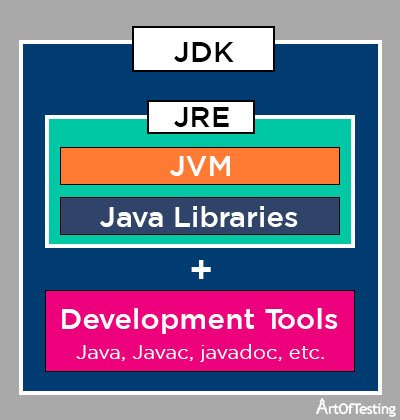
Ques.5. What is Javac?
Ans. The compiler used in Java is called Javac. The compiler itself comes inside the JDK. The purpose of it is to convert the Java program code line by line into bytecodes for the JVM to understand. The Javac can either be invoked by typing ‘Javac Filenamesaved.Java’, or by using the Java compiler API like the one in Eclipse IDE.
Ques.6. What is JRE?
Ans. JRE is Java Runtime Environment. The JRE is the platform that provides the environment for the Java programs to run (keep in mind: to run and not develop). It is the top layer that is used by us, the end-users.
It consists of the JVM + library classes + supporting tools and is part of the JDK. The programs are run on JRE as it loads the classes, verifies the access to the memory, and retrieves system resources.
Ques.7. What is JVM?
Ans. The JVM (Java Virtual Machine) is the interpreter and is the core of both JDK and JRE. The JVM verifies and executes the program line by line once the program is converted into Bytecode. It consists of a class loader. Memory area, execution engine, JNI, and Native method libraries.
Ques.8. Why is Java considered platform-independent?
Ans. Java is called platform-independent, as the code compiled in Java can run on all OS irrespective of on which it was created. The code created in Java is compiled into an intermediate language called the Bytecode, which is understood by JVM and converted into native machine-specific language based on which OS it is being run on.
The other languages like C are saved in .exe format and are compiled directly into machine-specific language according to the OS being used. This means a code compiled on Microsoft OS cannot run on Linux OS.
However, the code in Java is first converted into an intermediate Bytecode language therefore if the OS supports the JVM the code can be executed easily (remember, Java is platform-independent but the JVM is not).
Ques.9. What do you mean by JIT?
Ans. The JIT or the Just In Time is a compiler, part of the JVM which has two purposes-
- It converts the Bytecode into the native machine language.
- Optimize and run the code in the least possible time. i.e. boosts the performance by creating less load on the computer’s compiler.
Thus the inclusion of JIT enhances the performance of the application.
Ques.10. What is a classloader?
Ans. During runtime, a classloader loads .class files into the JVM(Java Virtual Machine). These classes can either be the System classes that come with Java or the classes created in our program.
Classloader belongs to Java.lang package and helps in loading classes only when required by the application(dynamically).
Ques.11. What are the different access modifiers in Java?
Ans. Access modifier is a frequently asked core Java interview question.
An access modifier defines the accessibility to a particular class, method, constructor, or field. There are 4 access modifiers in Java based on the type of access they provide. These are-
- Default – This modifier is applicable if no other modifier is specified. This means that the access level is within the current package and cannot be accessed outside the package.
- Private – Access is within the current class and not outside of it.
- Protected – The access within the current package unless a child class is created outside the package. It can be accessed through a child’s class.
- Public – As the name suggests there is no restriction. It can be accessed from within the class/package or outside the class/package.
Ques.12. What is OOPS?
Ans. OOPS stands for Object-Oriented Programming system. OOPs is a concept of instantiating a class by creating an object. The object contains data and methods. The OOPs concept is used to increase the flexibility of the program and make it look clean.
Ques.13. What are the four pillars of OOPS?
Ans. Abstraction, encapsulation, polymorphism, and inheritance are the 4 pillars of OOPS.
Ques.14. What is an Object in Java?
Ans. An Object is an instance of the class, created to store data and methods. An object has its identity, state/attribute, and behavior. For example, in a class named Animals an object dog is created. It has attributes like its breed, color, etc. It has behavior barks, runs, eats, etc.
Ques.15. Explain classes in Java.
Ans. A class is a collection of objects, that acts as a blueprint or template. It is not a real-world identity and therefore, also does not occupy space. A class named animal can contain objects named dog, cat, horse, etc. with methods like eat, run, sleep, etc. Therefore, a class contains fields, constructors, methods, nested class, and interface.
Ques.16. Can we have an empty filename in Java?
Ans. Yes, a Java file can be kept empty. Just save it by ‘.java’ and compile by entering the command Javac ‘.java’.
Ques.17. What are constructors in Java?
Ans. A constructor is a method that is invoked when a class is instantiated and memory is allocated to the instance. When the new keyword is used to create an object, the constructor is called. The constructor should have the same name as the class being instantiated.
Ques.18. What are the different types of constructors?
Ans. There are two types of constructors-
- Default constructors – These constructors are created by the compiler itself when no constructor is defined in the program. These constructors have no parameters, so the instances are initialized with default values.
- Parameterized constructor -These constructors are used to pass certain values/arguments to the objects created.
Ques.19. Can a constructor be overloaded?
Ans. Yes, a constructor can be overloaded. This works the similar way the overloading methods work, the number of arguments or the data type of the parameters are changed to overload.
Ques.20. Can constructors be inherited?
Ans. No, a constructor cannot be inherited. However, a constructor of a superclass can be invoked by the subclass.
Ques.21. What is the difference between constructors and methods?
Ans. Constructors-
- It is invoked when a class is instantiated, to initialize the state of the instance.
- They are a type of special method and don’t have a return type.
- The compiler provides a default constructor if there is none mentioned in the program.
- The name of the constructor must be of the same name as the class instantiated.
Methods-
- The behavior of the object is reflected by the method.
- A method has a return type.
- No default method is created by the compiler if none is mentioned in the program
- A method can or cannot be the same as the name of the class.
Ques.22. What is a wrapper class in Java?
Ans. To include primitive data types like int, boolean, char, etc. In the family of objects(as Java is object-oriented) wrapper class is used. For every primitive data type, we have a wrapper class.
For example – Integer for int, Float – float, Character for char, etc.
Ques.23. What is a package in Java? What are its advantages?
Ans. A package in Java is a type of file directory or folder that contains all the related classes, sub-classes, and interfaces. There are 2 types of packages in Java, built-in and user-defined. One such built-in package is ‘java.lang’ imports the lang package which has all the fundamental classes required to create a basic program.
Ques.24. What are global, local, and instance variables?
Ans. A global variable is declared at the start of the program, within the class, and is accessible by all the parts of the program.
A local variable is created/declared inside a method, and cannot be accessed outside that.
An instance variable is associated with an object and is declared within a class but outside the method. All the objects of that class can create their own copy of that variable with their own value associated with it.
Ques.25. What is the static keyword in Java?
Ans. The concept of static keywords is one of the commonly asked core Java interview questions.
The static keyword associated with any method, variable, or nested class means it belongs to the class and not any instance of the class.
Ques.26. What do you mean by a static method?
Ans. A static method is a method for which there is no requirement to make an object. They can be invoked without creating an object. Therefore they belong to the class and not to the instances of the class.
Ques.27. What is an instance method?
Ans. An instance method requires an object of the class to call or invoke it. Therefore, they belong to the object of the class and not the class itself.
Ques 28. What is a string in Java?
Ans. A string is a sequence of characters and comes under non-primitive data types. A string is a class in Java and extends the object class of Java. It is used to manipulate strings in the program, through various methods included in it. Syntax-
String s= “name”; or String s = new String();
Ques.29. What is a string constant/literal pool?
Ans. string constant pool is a special memory location that stores string objects, or it is a place in the heap memory which stores string literal values.
Ques.30. What are the different classes to create strings? Differentiate between them.
Ans. There are 3 classes to create strings-
- String – Strings created through string class are immutable i.e. they cannot be altered once created.
- String Builder – Strings created through this are mutable(changeable) and preferable when used from a single thread.
- String Buffer – Strings created through this are mutable, i.e. the values can be changed, and also it is thread-safe. This means it can be accessed by multiple threads and yet will remain safe.
Ques.31. Why are strings immutable in Java?
Ans. This is one of the most frequently asked core Java interview questions. Immutable means something that cannot be modified. So, when we say that String is immutable in Java. It means String objects cannot be modified once created. In case we try to change the value of the String then a new object will get created.
Now, let’s see the benefit of making String immutable. Strings are immutable in Java because it uses the concept of string literal or string constant pool.
If a new String object is created with the value – “ArtOfTesting” then the same will be placed in a Java heap memory called String pool. Now, whenever new String literals are created with the value – “ArtOfTesting” then instead of creating multiple objects, each object will point to the same value (i.e. “ArtOfTesting”) in the String pool.
This saves a lot of memory. If the String value in Java is allowed to be modified then the concept of the String pool will not hold. This is because, changing the value of one object can update the value of other objects also, which may not be desired.
Ques.32. Why is string final in Java?
Ans. A String is made final to maintain its property of immutability thus saving it from any changes.
Ques.33. Difference between == and .equals() method in Java.
Ans. == operator is used to compare two strings when string variables are pointing to the same memory location(address reference). The .equals() is used to check the object values(content comparison).
Ques.34. What is inheritance?
Ans. Inheritance is one of the concepts of OOPS where one class acquires/inherits the properties of the parent class. This ensures the reusability of methods and fields created in the parent class into the new classes created in the program. Syntax-
//A is sub-class and B is parent class
Class A extends Class B{
//code with methods and fields
}
Ques.35. What is polymorphism?
Ans. Polymorphism means taking many forms or shapes. In Java, polymorphism occurs when multiple child classes inherit the methods of a parent class. For example, a super class named vehicles with method wheels(); will have sub-classes such as Car, Scooter, etc. Each of these will have its own implementation of the method wheels.
Ques.36. What is Abstraction?
Ans. Abstraction is a method to hide data in Java. The purpose is to create an abstract method that is used to hide the implementation or the working of the program and show the only necessary information.
Its real-life example would be a car. Only the steering wheel and indicators are visible but the internal working is hidden.
Ques.37. What is encapsulation?
Ans. Encapsulation is a way in Java to wrap variables and methods acting on those variables in a single block. In this, variables declared in the current class are inaccessible by the other classes and can only be accessed by the (getter and setter) methods of the current class. Consider a capsule, the medicine inside it is hidden from the patient and appears to be plastic from the outside.
Ques.38. What is the main difference between encapsulation and abstraction?
Ans. The major difference between encapsulation and abstraction is that abstraction is used to hide unwanted or unnecessary information. This can be done by using abstract classes and interfaces.
The encapsulation is used to hide data as a single block with all the variables and methods inside it. The data can be accessed through getter and setter methods.
Ques.39. What do you mean by the interface in Java?
Ans. An interface is a collection of abstract methods in Java. A class can implement the interface by using the ‘interface’ keyword, which will result in inheriting all the abstract methods present in the interface. The interface provides complete(100%) data hiding.
Ques.40. What are the different types of inheritances in Java?
Ans. There are 4 usable inheritance types in Java-
- Single-level inheritance – Just one child class inheriting the properties of a parent class.
- Multi-level inheritance – Multiple child classes inheriting properties of a multiple parents class. i.e. Class A extends Class B and Class C extends Class B.
- Hierarchical inheritance – When many child classes have a single parent class.
- Hybrid inheritance – As the name suggests it is a mix of two or more types of inheritance.
Ques.41. What are method overloading and method overriding?
Ans. Both methods are involved when the code is based on polymorphism. The method overloading is static polymorphism and the method overriding is dynamic polymorphism.
Method overloading-
Method overloading is a part of polymorphism where a class has multiple methods with the same name but different arguments. Conditions for creating method overriding are-
- Methods should have the same name.
- Arguments passed should be different (number, type, sequence).
- Methods should belong to the same class.
Example-
Class Overload{
void show(){
System.out.println("sum");
}
void show(int a){
System.out.println("multiply");
}
public static void main(String args[]){
Overload t = new overload();
t.show();
t.show(10);
}
}
Method overriding–
Creating and implementing a method with the same name in a subclass as the parent class is called method overriding.
- The methods’ name should be the same
- Arguments passed should be the same(number, type, sequence)
- Methods should belong to different classes
- Creating IS-A relationship.
Example-
Class Example1{
void show(){
System.out.println("method1");
}
}
Class Example2 extends Example1{
void show(){
System.out.println("method2");
}
public static void main(String args[]){
Example1 a= new Example1();
a.show(); //method1
Example2 b= new Example2();
b.show(); //method2
}
}
Ques.42. What are the base class and derived class?
Ans. This is a concept of inheritance in OOPs. a base class is also called the parent class, from which the other classes derive. A derived class or the child class inherits properties or functions from the base class.
Ques.43. What are the limitations of inheritance?
Ans. The limitations of inheritance are-
- The parent and the child classes become tightly coupled.
- Needs proper and careful implementation due to multiple closely associated classes.
- Increases execution time due to jumping between the parent and child classes.
- Modifications have to be done to both parent and the child’s class.
Ques.44. Can instances of an abstract class be created?
Ans. No, abstract classes cannot be instantiated. This is because of the abstract methods which are empty/without a body. They act as a base for subclasses. They have to be extended and built upon.
Ques.45. Why does Java not support multiple inheritances?
Ans. Implementing multiple inheritances creates complexities like the diamond problem. The diamond problem occurs when ambiguity occurs between two classes which will be overridden. For example, class A has subclasses class B and class C. B&C are superclasses to class D. A method in A gets overridden by both B&C. Then from which class will D inherit this method? Such complexity is called a diamond problem.
Ques.46. What is aggregation in Java?
Ans. Two objects have two types of relationships between them, IS-A and HAS-A. The aggregation is a HAS-A relationship which means it is a one-way relationship when two objects are involved.
For example, two classes are declared, Student and Address. A student will have an address, but it’s not true vice-versa. That means an address does not need to have a student. This is a HAS-A relationship.
Ques.47. What is composition?
Ans. Composition is a part of aggregation and reflects a part of a relationship. It is the restricted form of relationship of aggregation, where the two objects related are highly dependent on each other, i.e. they cannot exist without each other.
Suppose there is a college with many departments. If the college is closed then there will be no meaning for the individual departments.
Ques.48. What are virtual functions in Java?
Ans. All the functions in Java are virtual functions(by default). There is no ‘virtual’ keyword in Java like in C++.
Ques.49. Can an abstract method exist without an abstract class?
Ans. No. If an abstract method is declared, an abstract class must exist. However, the vice versa is not true.
Ques.50. What are some advantages of OOPS?
Ans. The advantages of OOPS are-
1. Code maintenance – It helps in maintaining and modifying the code in the simplest way.
2. It helps in creating a clean structured program or code.
3. Reusability – You can inherit the same methods in different classes by using inheritance.
4. It is helpful for security purposes as it helps in data hiding.
Also, check 👉 OOPS Interview Questions
Ques.51. What is a super keyword?
Ans. The super keyword points to the instance of the super class or the parent class. It is majorly used to eliminate the confusion between the super and subclasses with same-name methods.
Ques.52. What is this keyword?
Ans. The ‘this’ keyword is used to point to the current instance of a method or a constructor. The keyword is mostly used to eliminate the confusion of multiple methods of the same name existing in the program.
Ques.53. What are the uses of this keyword?
Ans. The uses of this keyword are-
- Can be used as a reference variable to point to the instance of the current class.
- Can be used to invoke a constructor of the current class.
- It can be used to invoke the current class method.
- Can be passed as an argument in any method call.
Ques.54. Can this keyword be used to refer to static members?
Ans. A static member is called without creating an instance, and this keyword is used to refer to the current class object. Therefore, the answer is no.
Ques.55. What is constructor chaining?
Ans. The method of calling one constructor of the class using another constructor of the same class is called constructor chaining. It proves helpful when you want to perform multiple tasks through a single constructor instead of creating constructors for each task. It makes your code more readable.
Ques.56. How is constructor chaining achieved? Why is it done?
Ans. Constructor chaining can be achieved in two ways-
- By using the ‘this’ keyword for the constructor in the same class.
- By using the ‘super’ keyword for the constructor in the base/parent class.
It is done so as to make code easier. This is because initialization is done only once at a single place and parameters are passed all over the constructors in the program.
Ques.57. What is the init method in Java?
Ans. The init() method in Java is used for initialization purposes. It is an instance initialization method and is used by the JVM. Whenever a constructor is written in the program, the JVM considers its init method. This method is used by the compiler and therefore, not for the main program use purposes.
Ques.58. What is the actual superclass in Java?
Ans. The object class is the superclass of all the classes existing in Java.
Ques.59. Why are pointers not supported in Java?
Ans. Pointers are used to point to the memory of another variable. By not supporting pointers Java achieves security by abstraction as the pointers directly lead to the memory of a variable.
Ques.60. What is type casting in Java?
Ans. Type casting is a method to convert one data type to another data type in a program. This can be done manually by the developer or automatically by the compiler. Example-
int a = 45.66;
double d = (double)a;
Ques.61. What are the different types of type casting?
Ans. There are two types of typecasting-
Narrow Typecasting is also called explicit casting or casting down. It is the method of converting a larger data type into a smaller data type. Like, double-> float-> long-> int-> char-> short-> byte.
Widening Typecasting is also called implicit casting or casting up. It is the method of converting a lower data type into a higher data type. Like, byte-> short-> char-> int-> long->float->double.
Ques.62. What are some advantages of polymorphism?
Ans. Some advantages of polymorphism are-
- Helps in code reusability as the classes once written can be implemented again and again.
- Single variables can store multiple data types.
- Reduces coupling of classes, unlike inheritance.
Ques.63. What are some advantages of abstraction?
Ans. Some advantages of abstraction are-
- Helps in creating a secured program as only required data is visible.
- Reduces the complexity of the program.
- Easier implementation of the software.
- Groups the related classes as siblings.
Ques.64. Can we overload the main() method?
Ans. Yes, a main method can be written several times in a code. The catch is, you have to call the overloading main() method from the original default main() method of the program.
Ques.65. What is the final keyword in Java?
Ans. The final keyword added to any entity will declare it permanent. No changes can be made to the value once it is declared final. They can neither be overridden nor inherited. The final keyword is a non-access modifier.
Ques.66. Can the main method be declared final?
Ans. Yes, the main() method can be declared final. Most methods are declared final so that they do not get overridden.
Ques.67. Can we declare an interface as final?
Ans. The purpose of the interface is to provide methods that can be implemented. The final keyword abstains a method from being inherited or implemented. Therefore, an interface can never be final.
Ques.68. What is static binding and dynamic binding?
Ans. Identifying classes and objects during compile time is called static binding. Methods like private, static, etc. are identified earlier because they cannot be modified or overridden.
Whereas, late binding also known as dynamic binding is when the type of the object is identified during run time. Method overriding is a perfect example of dynamic binding.
Ques.69. What is the InstanceOf operator?
Ans. The InstanceOf is a keyword that checks if the object created is an instance of the mentioned class or subclass.
Ques.70. What do you mean by a multithreaded program?
Ans. Execution of multiple lines of instructions(threads) simultaneously so that multiple tasks can be performed at the same type is called multithreading.
Ques.71. What is exception handling?
Ans. Exception handling in Java helps in recognizing errors in the program. This ensures that the flow of the program is maintained even after an error is detected.
Ques.72. What are the types of exceptions in Java?
Ans. There are two types of exceptions checked and unchecked.
Checked exceptions are handled during the compile time. These include SQL exceptions, IO exceptions, etc.
The unchecked exceptions are those which cannot be checked or handled during compile-time and therefore throw an error during run time. These include ArrayIndexOutOfBoundsException, NullPointerException, etc.
Ques.73. Explain Java exception classes hierarchy.
Ans. The Java exception hierarchy starts from the throwable class which is a superclass. It is further divided into ‘exceptions’ and ‘errors’ classes. Errors are detected by the JVM. Some common errors are – OutOfMemoryError, unknown error, etc. Whereas exceptions are further bifurcated into checked and unchecked exceptions.
Ques.74. What is the ‘finally’ block?
Ans. The ‘finally’ keyword is used with the statement of the program that has to run even if an exception is thrown or not, i.e. important codes. It is used with the try and catch blocks. There is always one final block in the end.
Ques.75. Difference between throw and throws keyword.
Ans. When a program is unable to produce the required output, the ‘throw’ keyword is used. It helps us to create an exception and interrupt the flow of the program.
The ‘Throws’ keyword is used to signal a probable exception in a program that may occur when a method called is executed.
Ques.76. Can you catch multiple exceptions?
Ans. Yes, multiple exceptions can be caught in a program.
Ques.77. What is the difference between an exception and an error?
Ans. Exceptions can be handled with the try-catch blocks but errors cause disruption in the flow of the program and cannot be fixed by itself. Errors occur during the run time of the program. Most of the unchecked exceptions are errors only.
Ques.78. What do you mean by OutOfMemoryError?
Ans. When the JVM runs out of heap memory it throws an error called OutOfMemoryError.
Ques.79. Can you write a custom exception in Java?
Ans. Yes, we can write custom exceptions by creating a whole new class that ends with the name ‘Exception’.
Ques.80. What are some advantages of exception handling in Java?
Ans. The advantages of exception handling are-
- Separates the error handling codes from the main program code.
- Grouping the errors thus helping in solving them more quickly.
- Maintains the normal flow of the program.
Ques.81. How does exception handling work in Java?
Ans. We can define the exception-handling process as-
- Step1: An object of the error is created when an error is detected in the program. It is called an exception object and contains all the information of the error.
- Step2: Call stack methods are called to handle the exception.
- Step3: Particular code in the call stack is searched to find a way to handle the exception.
- Step4: That exception handler is chosen so as to catch the exception.
Ques.82. Explain 5 keywords used in exception handling.
Ans. Try – Exception is handled by writing the code inside the try block that might throw an exception.
Catch – The exception handling code is written in the catch block.
Throw – It is used by the user to create an exception if the code does not run in the desired way.
Throws – When we are aware of the checked exceptions and let the caller program know about those, the throws keyword is used before that exception.
Finally – This block always gets executed even if an exception is thrown. It is used with the try-catch blocks.
Ques.83. What is the chained exception?
Ans. When one exception explains the cause of the previous exception it is called a chained exception. For example, if you divide a number with zero, it will throw an Arithmetic exception. But the underlying cause is that it was an I/O exception and the program needs to know that. This is a chained exception.
Ques.84. What is a stack trace?
Ans. A stack trace is used to list all the methods and names of the classes that have been called or used till the time exception occurred. The stack trace helps in debugging the code.
Ques.85. Can a child class that is overridden throw an exception?
Ans. If the parent class does not throw any exception it is unlikely that the child class will throw one. But an unchecked exception can be thrown by it during run time regardless of whether an exception is thrown by the base class or not.
Ques.86. What is a nested class?
Ans. A class created inside another class or interface is a nested class. The method of nested classes is used to group similar classes together so that the code looks neat and is maintainable.
Ques.87. What are some advantages of nested classes?
Ans. Advantages of nested class-
- Helps to maintain the program neat and readable
- Code optimization
- Creates a special relation among the nested classes which gives access to the outer class members, including the private class.
Ques.88. What is the interrupt() method in Java?
Ans. The interrupt() method in Java throws InterruptedException whenever a thread is in a sleep or waiting state. There are 3 ways in which a thread can be interrupted-
- public void interrupt()
- public static boolean interrupted()
- public boolean isInterrupted()
Ques.89. What are the different ways in which strings can be compared?
Ans. We can compare strings in the following ways-
1. .equals() method
2. Using == operator
3. s.charAt() method
4. compareTo() method
5. .equalsIgnoreCase() method
6. compareToIgnoreCase() method
Ques.90. What are identifiers in Java?
Ans. Identifiers in Java are names given to a class, method, package, variable, etc. to ensure they can be identified easily. However, you cannot name them in any random way. There are some rules to create identifiers. Such as-
- Spaces cannot be used
- Special symbols cannot be used except underscore and $ sign.
- Reserved keywords of Java cannot be used.
- Integer values can only be used after 1st character.
Ques.91. What are the memory areas allocated by JVM?
Ans. There are 5 memory types in JVM-
- Heap – Memory allocation for objects
- class(method) area – For each of the classes’ structures(methods, variables, etc.)
- Stack – Holds local variables, methods invoked, methods returned, and partial results.
- PC(program counter) – Holds the address of the current instruction being executed.
- Native method stack – Stores all the native methods used.
Ques.92. What is synchronization in Java?
Ans. When multiple threads(instructions) try to access the same resource, errors are bound to happen. Using synchronized blocks in Java, you can control the access to these multiple threads. This is called synchronization. These synchronized blocks can be identified by the synchronized keyword.
Ques.93. Does the order of specifiers matter while creating a method?
Ans. No, the order does not matter till every specifier is mentioned. Public static void is the same as a static public void.
Ques.94. Do local variables have a default value?
Ans. No, default variables do not have a value until initialized. The same goes for primitives and object references.
Ques.95. What are the restrictions to the static methods?
Ans. The different restrictions in the static method are-
1. A static method cannot call a non-static method directly nor use a non-static data member.
2. This and super keywords cannot be used inside a static method.
Ques.96. Can a program be run without the main method?
Ans. Yes, it is possible by using a static block.
Ques.97. What is the difference between Array and ArrayList?
Ans. Array-
1. It is a dynamic object and holds similar values.
2. It is static in size, meaning the size cannot be manipulated once created.
3. Can store both objects and primitives.
4. Multidimensional.
ArrayList-
1. It is a class of Java collections framework and comes under Java.util package.
2. It is dynamic in size. Therefore, can be resized according to the need.
3. Cannot store primitives.
4. It is always of a single dimension.
Ques.98. What is a list in Java?
Ans. The list is an interface in Java in which objects can be stored in an ordered way(indexed) and duplicate and null values can also be stored. ArrayList, Linked List, vector, and stack are implementation classes of the List.
Ques.99. What is the Collection interface in Java?
Ans. It is a framework that acts as a base to store and manipulate groups of objects. Classes like ArrayList, LinkedList, Vector, and interfaces like queue, list, and set come under it.
Ques.100. What is a hash map?
Ans. Hashmap is an implementation of a map interface. The data is stored in pairs in the form of a key, value. The key acts as an index to another object(value). The objects stored can be retrieved in the shortest time (O(1)) if the key is known.
This article on core Java interview questions is shared by Kanika Rawat. She is a tech enthusiast and has a keen interest in coding.
please next time give me the examples
Try to add basic java programs also for better practise .
Implicit and explicit casting is wrong
Implicit casting is also called as widening is actual answer.
Ques.61. What are the different types of type casting?
The answer to the above question is wrong, please review it.
Ques.61. What are the different types of type casting?
Expected:- Narrow Typecasting is also called Explicit casting or casting down,
and the given example is also wrong.
Thanks, must have been a typo. Corrected now.