In this article, we are going to learn the Java basics or the core concepts of Java programming. These concepts are absolutely necessary to be able to write or understand any kind of Java program.
In our previous article, we learned about Java download & setup and created our first hello word program. Now, is the time to understand it.
Content
Understanding the HelloWorld Program
public class HelloWorld {
public static void main(String args[]) {
System.out.print(“Hello World”);
}
}
- Every statement in Java must be inside a class. In our case, it is “HelloWorld”. Also, the name of the file should be the same as that of the class name, i.e. HelloWorld.java.
- Every Java program must have a main() (read as the main function) and the execution of the program starts from here. The String args[] in Java is an array of strings that stores arguments passed by the command line while executing the program. Right now it is not important to exactly know how it works.
- We use the print() to display something on the screen during the execution of the program. The println() method does the same except that it automatically moves the cursor to the next line after printing.
- Java program’s main method has to be declared static because keyword static allows main() to be called without creating an object of the class. If we omit the static keyword before the main(), the Java program will successfully compile but it won’t execute.
Simple Java Program
Let us begin with learning the basic terms by considering an example-
//sample Java program to find the product of two numbers
public class Example {
public static void main (String args[]) {
int x = 5;
int y = 2;
int product = x * y;
System.out.println("Product = " + product);
}
}
Variables
Also known as identifiers, they are defined as a location in the memory where the values are stored. It has a name, a type, and a value. In the above example, the variables are x, y, and product.
Keywords
These are the words that have special meaning in Java programming. For example – int, main, public, static, void, etc are all keywords. There are a number of keywords in Java and you can easily find them on the internet. Using keywords as identifiers leads to errors.
Datatypes
As the name suggests, datatypes are the types of data that a value can possess. There are two types: Primitive/Built-in datatypes and User-defined datatypes. The primitive datatypes in Java along with their memory size are byte(1 byte/8 bits), short(2 bytes), integer(4 bytes), long(8 bytes), float(4 bytes), double(8 bytes), character(2 bytes), boolean(1 bit). The User-defined datatypes are class, object, string, array, interface. We will be learning about each of them in detail later. In the above example, the datatype used is int(integer).
Operators
Operators in Java help in performing different types of operations. There are seven types of operators-
- Arithmetic operators – They help in performing arithmetic operations. They require two operands. Arithmetic operators are +, -, *, /, % (MOD).
- Relational operators – They are also known as comparison operators and help in comparing any two values. In Java, relational operators are >, >=, <, <=, ==, !=.
- Logical operators – They are used to perform operations on logical combinations of boolean (true/false) values. They are binary operators used on two operands. The logical operators used in Java are &&(AND), ||(OR), !(NOT).
- Assignment operators – These operators are used to assign values of an expression to a variable. Under this, there is a shorthand operator. For example, x += 5 is the same as x = x + 5. The assignment operators in java are =, +=, -=, *=, /=, %=.
- Increment and decrement operators – These operators are used for increasing or decreasing the value of a variable by 1. They are of 2 types: pre and post. Pre-increment (++x) or pre-decrement (–x) first increases or decreases the value by 1 then uses the value of the variable respectively. Whereas in the case of post-increment (x++) and post-decrement (x–), the value of the variable is first used and then increased or decreased by 1. These operators work only on one operand.
- Bitwise operators – These are for operations on data at the bit level. In Java, there are various bitwise operators. Some of them are: & (AND), | (OR) , ^ (NOT), << (Left Shift), >> (Right Shift), etc.
- Ternary operators – These are also known as conditional operators and require three operands. The syntax of the ternary operator is-
value_variable = (condition) ? expression1 : expression2;
where expression1 will be evaluated if the condition is true and expression2 will be evaluated if the condition is false.
An example is-
char result = (marks>=40) ? ‘P’ : ‘F’;
Conditional Statements
Conditional statements are used when we want to evaluate certain expressions based on a condition. For example, if we want to find out whether a person is an adult or not based on his/her age, then we need to use conditional statements. The different kinds of conditionals are-
1. if else
When we want to check different conditions and perform different sets of instructions, we use this conditional statement. For example, if we want to find the grade of a student based on his marks then-
int marks = 87;
char grade;
if (marks >= 85 && marks <=100) {
grade = 'A';
}
else if (marks >=70 && marks <85)
grade = 'B';
else if (marks >=50 && marks <70)
grade = 'C';
else if (marks >= 35 && marks < 50)
grade = 'D';
else
grade = 'F';
Notice that I have used the logical AND (&&) operator to express a range in the condition. Also, if the body of the conditional statement is just one line long, then the curly brackets are optional. Otherwise, they are mandatory.
The last condition must not be written with ‘else’ statement. It implies the values that haven’t matched with any of the above conditions. We can also use only ‘if’ without ‘else’ or we can use only one ‘if’ and one ‘else’ depending on the number of conditions and instructions.
2. Switch case
It is a multiple branch structure that is used to execute instructions where we can consider only specific conditions. An example for finding the day of the week based on a digit between 1 to 7 starting from Sunday is-
int day = 2;
switch(day) {
case 1: System.out.println("Sunday");
break;
case 2: System.out.println("Monday");
break;
case 3: System.out.println("Tuesday");
break;
case 4: System.out.println("Wednesday");
break;
case 5: System.out.println("Thursday");
break;
case 6: System.out.println("Friday");
break;
case 7: System.out.println("Saturday");
break;
default: System.out.println("Invalid Choice");
}
Switch…case is an alternative for if…else. But in switch case, we cannot use a range in the condition part and we can work with only integer or character values in switch case. The ‘break’ statement is known as a jump statement and is used to break and come out of the loop wherever it is faced.
If we do not use ‘break’ here, the next cases will also be executed until a break is encountered. The ‘default’ here is an alternative for the ‘else’ of if..else if..else structure. The switch case structure is usually used for menu-driven programs. Usually, a menu is presented to the user and the instructions are carried out according to the option chosen.
Loops
If we want to print numbers 1 to 100, then how can we do it? Surely we cannot write 100 statements printing a number each time. This is the reason we need loops. Loops help in iterating or repeating a certain set of instructions for a number of times. There are three types of loops in Java programming – For, While, Do…while. The syntaxes of each are-
For loop
for(initialization; test condition; update expression) {
Body of the loop;
}
While loop
initialization;
while (test condition) {
Body of the loop;
Update expression;
}
Do while
initialization;
do {
Body of the loop;
Update expression;
} while (test condition);
All three are performing the same function but just have different formats. For and While are entry-controlled loops. The do..while is exit controlled as the body of the loop is getting executed at least once even if the initial condition is false.
If the body of the loop contains only one statement then curly brackets are optional. Notice the use of semicolon after while in Do…while and its absence in While. Example of printing numbers 1 to 100 in all three formats are.
For loop-
int i;
for(i = 1; i<=100; i = i+1) {
System.out.println(i);
}
While loop-
int i = 1;
while (i<=100) {
System.out.println(i);
i = i + 1;
}
Do while loop-
int i = 1;
do {
System.out.println(i);
++i;
} while (i<=100);
The working of ‘for’ loop is according to the below steps:
- Initializing the variable ‘i’ to value 1;
- Checking for the test condition. If the condition is satisfied, then the body will be executed.
- After it reaches the end of the body, it will move up to the update expression. After that, it will again check the test condition. If true, then the body will be executed. If false, then it will come out of the loop.
There is one more For-each loop in Java, for better understanding, we will explain it later in this article after studying Arrays.
Jump Statements
There are two jump statements in Java-
1. Continue – This statement is used when we want to terminate a particular iteration of a loop. For instance, in the above loop example, if we do not want to print those numbers which are multiples of 5 then we use continue like.
int i;
for(i = 1; i<=100; i = i+1) {
if (i % 5 == 0)
continue;
System.out.println(i);
}
2. Break – This statement is used when we want to terminate the entire loop and come out of it. We already saw this statement is used in the switch…case. It can also be used in other conditional statements.
Methods
Methods are basically blocks of code inside curly brackets belonging to a method name which makes the program modular and simpler. The syntax of a method in Java is-
Access_specifier return_type method_name (arguments) {
Body of the method;
}
Let us understand these terms.
Access_specifier: They set the accessibility of the method in the Java program. They are also known as access modifiers. There are four types of access specifiers in Java: public, private, protected and package. We will learn about these in the next article.
Return_type: It is the data type of the value returned by the method. Yes, a method can return a value. If the method does not return any value, then the return type becomes ‘void’.
Method_name: It is the name of the method which is being defined and will be called. Arguments/Parameters: These contain the values passed on to the method which will be used by the method. They are variables accompanied by their datatype.
A function or method has 3 parts-
1. Function declaration
Often we will have function declared without having a body, like this-
public int sum(int, int);
2. Function definition
The function along with its body is known as the definition of the function. These are usually written outside the main(). Example-
public int sum (int a, int b) {
int sum = a + b;
return sum;
}
3. Function call
Now that we have written the declaration and definition of the function, it is time to call the function. A function is not going to be executed unless it is called explicitly. So, the function calling statement is present inside the main(). The function call statement for the above example is-
int s = sum(5, 10);
Since the method is returning an integer value, it has to be stored in an integer value when it is called.
Working of a method
The function call is the first step in the execution of a function. In this example, the values 5 and 10 will be assigned to ‘a’ and ‘b’ respectively. After this, the program will start looking for the function definition above the point where it has been called. If it finds a function declaration, then it will start searching the whole program for the definition.
Then the body of the function will be executed and control will return back to exactly the point after the function call. The return statement in a function body is the terminating statement in the function and no statement after it will be executed. If there is more than one return statement, only one of them will be executed.
Method overloading is allowed in Java. It means defining many methods with the same method name but different arguments. Java automatically chooses the function with the matching parameters.
Functions inside a class are known as methods. But every line of code in Java is written inside a class. Thus, all the functions in Java are a method.
Arrays
An array is a set of similar values stored in consecutive memory locations.
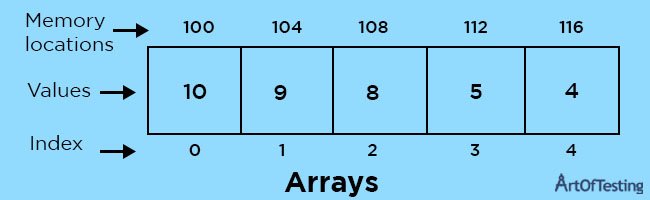
As the above picture shows, it is an array of 5 elements of type integer having index values from 0 to 4 and consecutive memory locations from 100 to 116 with 4 bytes occupying each element since integer occupies 4 bytes of memory. Arrays are very helpful in programming for manipulating data of similar type.
An example of creating the above array is – int[] age = new int[5];
Let’s quickly check, iteration through array element using loops-
int arr[]={10, 9, 8, 5, 4};
//Print array elements
System.out.println("Array elements-");
for(int i=0; i<arr.length; i++) {
System.out.println(arr[i]);
}
For each loop
Now that we have learned about arrays as well as loops, let’s look at something we can do by combining the two. When we want to specifically traverse an array, we use this loop. It starts with the same keyword ‘for’ but the syntax inside the round brackets changes a bit. The syntax is-
for ( data_type variable : array_name) {
Body of the loop using the variable;
}
An example of using for-each loop is-
// Finding and printing the odd and even numbers in an array
for (int n : arr) {
if (n%2==0)
System.out.println(num + "is even");
else
System.out.println(num + "is odd");
}
Notice how for-each loop is different from the normal for loop in terms of syntax as well as usage. For-each loop can become very handy when we only want to traverse and check any specific conditions for all the elements in an array from the leftmost element to the rightmost, in increasing order of 1.
Alright now, let’s move on to the class and object, which form the heart and soul of Java programming. We will study these in detail, in our next tutorial – OOPS in Java but let’s briefly learn about these here also.
Class
A class in Java is defined as a group of objects with some properties and relationships. As stated earlier, every Java program is inside a class. Thus, it is important to understand what class is. A class can also be defined as a blueprint or a template having certain attributes from which we create objects. For example, a car, chair, table, fruit, etc all are classes. The syntax of defining a class is-
access_specifier class className {
Class definition containing variables and methods;
}
An example of a class other than what we have used in our previous programs is-
public class Car {
String modelName;
String colour;
int mileage;
}
Object
An object is an identifiable, real-time entity with some characteristics and behaviour. An object is an instance of a class. A class can have multiple objects. An object can also be defined as a bundle of data and its behaviour. For example, modelName, colour, mileage are all objects/attributes of the class Car.
We usually create objects of a class in the main(). An example of creating an object of class Car in Java is-
Car obj = new Car(); //obj is our object of type Car
We will learn in detail about classes and objects in the next article.
Comments in Java
Comments are non-executable statements used in Java to increase the understanding and readability of the program and anyone else who reads it later. Java allows both single-line and multi-line comments. Example-
//This is single line comment
/* this is a
multi-line
comment */
Rules for writing a Java program
- Java always uses higher CamelCase for writing class names and lower camelCase for writing method names. Variable names should always start with either an alphabet or an underscore(_). It can contain digits but not at starting. Use meaningful names for variables.
- Java is case-sensitive. ‘Hello’ is not the same as ‘heLLo’.
- Use indentation for structuring your program. The body of a class definition and method definition should be indented to increase the readability of your program.
- Every executable statement in Java should terminate with a semicolon.
Conclusion
With this, we reach the end of Java Basics. We learned about variables, keywords, data types, different kinds of operators, conditional statements. We also learned when and how to use loops, methods in Java, Arrays and its structure, Class and Object in Java, comments, and the various rules and few conventions to be followed while writing a Java program.
I hope that you have understood these basics clearly but practice makes a man perfect and I suggest that you start practicing simple Java programs just to get into the habit of coding, to become familiar with the syntax of Java, and obviously to boost up your self-confidence.
Alright then, in the next article we will be learning the Object-Oriented concepts of Java Programming which form the major part of this language.