As a rule of thumb, each test method must be independent of other i.e. result or execution of one test method must not affect the other test methods. But at times in automation, we may require one test method to be dependent on others.
We may want to maintain dependency between the test methods in order to save time or for scenarios where the call to run a particular test depends on the test result of some other tests.
TestNG is a testing framework that provides us with two attributes- dependsOnMethod and dependsOnGroup within @Test annotation to achieve dependency between the tests. Now let’s explore these attributes.
Content
dependsOnMethod
Using ‘dependsOnMethod’ attribute within @Test annotation, we can specify the name of the parent test method on which the test should be dependent.
Some points to remember about dependsOnMethod-
- On execution of the whole test class or test suite, the parentTest method will run before the dependentTest.
- If we run the dependentTest alone even then the parentTest method will also run that too before the dependentTest.
- In case, the parentTest method gets failed then the dependentTest method will not run and will be marked as skipped.
@Test(dependsOnMethods={"parentTest"})

Code Snippet
In the below code snippet, we have two test methods – parentTest() and dependentTest(). The dependentTest method is made dependent on the parentTest method using dependsOnMethods attribute.
public class TutorialExample {
@Test
public void parentTest() {
System.out.println("Running parent test.");
}
@Test(dependsOnMethods={"parentTest"})
public void dependentTest() {
System.out.println("Running dependent test.");
}
}
Output
On running the dependentTest() alone, the parentTest() method will also run and that too before the dependentTest(). This is done to maintain the dependency.
Running parent test. Running dependent test. PASSED: parentTest PASSED: dependentTest =============================================== Default test Tests run: 2, Failures: 0, Skips: 0 ===============================================
dependsOnGroup
The dependsOnGroup attribute is used to make a test method dependent on a collection of tests belonging to a particular group. Thus ensuring that the dependent test will run after all the tests of the parent group are executed.
Some points to remember about dependsOnMethod-
- On execution of the whole test class or test suite, the tests belonging to the parent group will run before the dependentTest.
- If we run the dependentTest alone even then all the tests belonging to the parent group will also run that too before the dependentTest.
- In case, anyone or all of the tests of the parent group method gets failed then the dependentTest method will not run and will be marked as skipped.
@Test(dependsOnGroups = "groupA")
Code Snippet
In the below code snippet, we have two test methods – testMethod1ForGroupA() and testMethod2ForGroupA() belonging to group groupA. Then we have a dependentTest method – dependentTestOnGroupA that is made dependent on the methods of groupA using dependsOnGroups attribute. For the sake of the demo, we are intentionally failing the testMethod2ForGroupA.
public class TutorialExample {
@Test(groups = "groupA")
public void testMethod1ForGroupA() {
System.out.println("Running test method1 of groupA");
}
@Test(groups = "groupA")
public void testMethod2ForGroupA() {
System.out.println("Running test method2 of groupA");
Assert.fail();
}
@Test(dependsOnGroups = "groupA")
public void dependentTestOnGroupA() {
System.out.println("Running the dependent test");
}
}
TestNG result
On running the dependentTestOnGroupA() alone or running the whole test class, we can observe the following output.
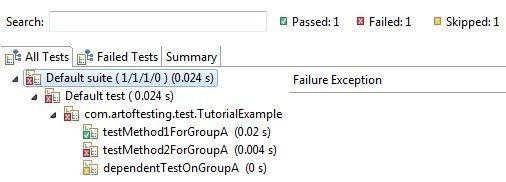
Here, we can observe that TestNG attempts to run all the 3 tests and because of the dependency, runs testMethod1ForGroupA and testMethod2ForGroupA first. Now, since testMethod2ForGroupA gets failed, so, the dependentTest i.e. dependentTestOnGroupA is not run and is marked as skipped.
Hello Kuldeep, amazing explanation!! It’s very well written and clear!
Just one note, I think in the last part, it should be: ” testMethod1ForGroupA and testMethod2ForGroupA” instead of testMethod1ForGroupB
Thanks, this is corrected now.